Vault7: CIA Hacking Tools Revealed
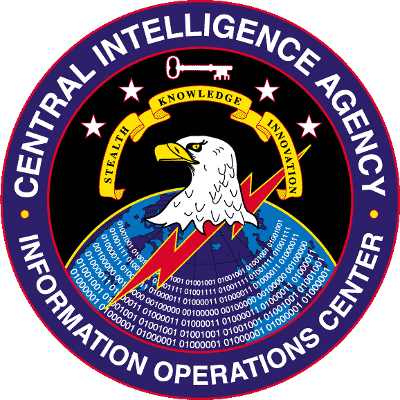
Navigation: » Directory » DART
Owner: User #1179751
Watch For PSP Popups
This guide is an example oh how to use the PSPPersonal Security Product (Anti-Virus) testing tools found in the AEDApplied Engineering Devision leafbag. The purpose of these classes are to give developers and testers a better idea as to what level of alert is made available to the user.
Step-by-step guide
We'll be filling this in later as we figure it out. This is a work in progress User #4194308 User #4194308
- Insert your steps.
- You can also copy and paste or drag and drop images into your instructions.
#This should seem familiar because it is required for all leafs
from datetime import datetime, timedelta
#This is a hack to allow us to enumerate the host object
import tybase.palantir.client
import tybase.undermine.client
import difflib
def read_log(path_to_log):
f = open(path_to_log, 'r+')
return f.read()
#Back to the familiar
class Kaspersky():
def __init__(self, host):
""""
Standard constructor, but must implement some basic functionality.
:param host - references the host object of the machine running the PSPPersonal Security Product (Anti-Virus) being tested.
Requirements: The constructor must do the following:
1. Implement self.path_to_psp - full path to the psp exe to be interacted with
2. Populate self.base_line - log dump at the beginning of the test, to limit data being presented to the
user.
"""
assert isinstance(host, tybase.palantir.client.Client)
assert isinstance(host, tybase.undermine.client.Client)
self.host = host
#This is where our files will be dumped to
self.outfolder = "C:\\tmp\\"
self.outfile = self.outfolder + "kasp.txt"
if not self.host.path_exists(self.outfolder):
self.host.execcmd("mkdir " + self.outfolder, shell=True)
#hard code the locations the av product could reside.
potential_path = ['\"C:\\Program Files\\Kaspersky Lab\\Kaspersky Internet Security 14.0.0\\avp.com\"', '2']
self.path = potential_path[0]
for p in potential_path:
if self.host.path_exists(p):
self.path = p
if self.path == '':
self.host.log.error('Failed to find avp.com, psp tests will all fail')
scan_command = self.path + " REPORT /R:" + self.outfile
self.host.execcmd("\"" + scan_command + "\"", shell=True, wait=True)
self.base_line = self.host.remotefunc(read_log, self.outfile)
self.host.execcmd("del " + self.outfile, shell=True)
def run_static_scan(self, files_to_scan=[]):
"""
Kicks of a static scan for the PSP, will pass a list of files to scan to the scanner then parse the logs to see
if any hits occurred.
:param files_to_scan: List of full paths to desired files to scan
:return: True - Scan conducted, no issues to report
False - Something went wrong, should be treated as a failure case
"""
return_value = True
bad_word_list = ['Total detected', 'Detected exact', 'Suspicions', 'Treats detected', 'Untreated',
'Disinfected', 'Quarantined', 'Deleted']
list_of_files = ""
for s in files_to_scan:
list_of_files = list_of_files + '\"' + s + '\" '
scan_command = self.path + " SCAN /R:" + self.outfile + " " + list_of_files
self.host.execcmd("\"" + scan_command + "\"", shell=True, wait=True)
scan_results = self.host.remotefunc(read_log, self.outfile)
self.host.log.info(scan_results)
split_scan_results = scan_results.split("\n")
for line in split_scan_results:
s = line.split("\t")
if len(s) > 1:
for word in bad_word_list:
if s[0].find(word) != -1 and s[1] != "0":
self.host.log.warning("Hit found! " + line)
return_value = False
self.host.execcmd("del " + self.outfile, shell=True)
return return_value
def check_dynamic_logs(self, max_allowed_line_entries=5):
"""
Checks the logs generated by a PSPPersonal Security Product (Anti-Virus) and looks for anything of interest. This log will be compared with the log
file collected during initialization (so declaration should be the first part of any test, this the last part)
and checks the diff only. There are two types of failures, if a dirty word like CRITICAL THREAT DETECTED or
similar is found, or the log grew in size greater than max_allowed_line_entries.
:param max_allowed_line_entries: Maximum number of lines a log can grow before being considered a failure case
:return: True - No issues to report
False - Something went wrong, should be treated as a failure case
"""
return_value = True
bad_word_list = ['Object (file) detected', 'Detected object (file)']
scan_command = self.path + " REPORT /R:" + self.outfile
self.host.log.info(scan_command)
self.host.execcmd("\"" + scan_command + "\"", shell=True, wait=True)
new_log = self.host.remotefunc(read_log, self.outfile)
self.host.execcmd("del " + self.outfile, shell=True)
split_base = self.base_line.split('\n')
split_new_log = new_log.split('\n')
#this will give us a list of added lines to the file
diff = difflib.unified_diff(split_base, split_new_log, fromfile='base_line', tofile='new_log', lineterm='')
lines = list(diff)[2:]
added = [line[1:] for line in lines if line[0] == '+']
self.host.log.info(added)
for line in added:
for word in bad_word_list:
if line.find(word):
self.host.log.warning("Hit found! " + line)
return_value = False
return (len(added) < max_allowed_line_entries) and return_value
def psp_is_updated(self, max_days_since_update=14):
"""
Checks the PSPs logs to see if the product was updated within the time period set by max_days_since_update
:param max_days_since_update: Maximum number of days since a PSPPersonal Security Product (Anti-Virus) has been updated.
:return: True - No issues to report (PSPPersonal Security Product (Anti-Virus) has been updated recently)
False - Something went wrong, PSPPersonal Security Product (Anti-Virus) should be considered outdated
"""
update_list = 'Time Finish'
command = self.path + " STATISTICS Updater"
self.host.execcmd("\"" + command + "\"", shell=True, stdout=self.outfile)
update_logs = self.host.remotefunc(read_log, self.outfile)
#self.host.log.info(stuff)
split_update_logs = update_logs.split("\n")
return_value = True
for line in split_update_logs:
s = line.split("\t")
if len(s) > 1:
if s[0].find(update_list) != -1:
updated_time = datetime.strptime(s[1], "%Y-%m-%d %H:%M:%S")
if datetime.today() - updated_time < timedelta(days=max_days_since_update):
self.host.log.info("Logs are up to date")
else:
self.host.log.warning("PSP is outdated")
return_value = False
self.host.execcmd("del " + self.outfile, shell=True)
return return_value
Related articles
('contentbylabel' missing)